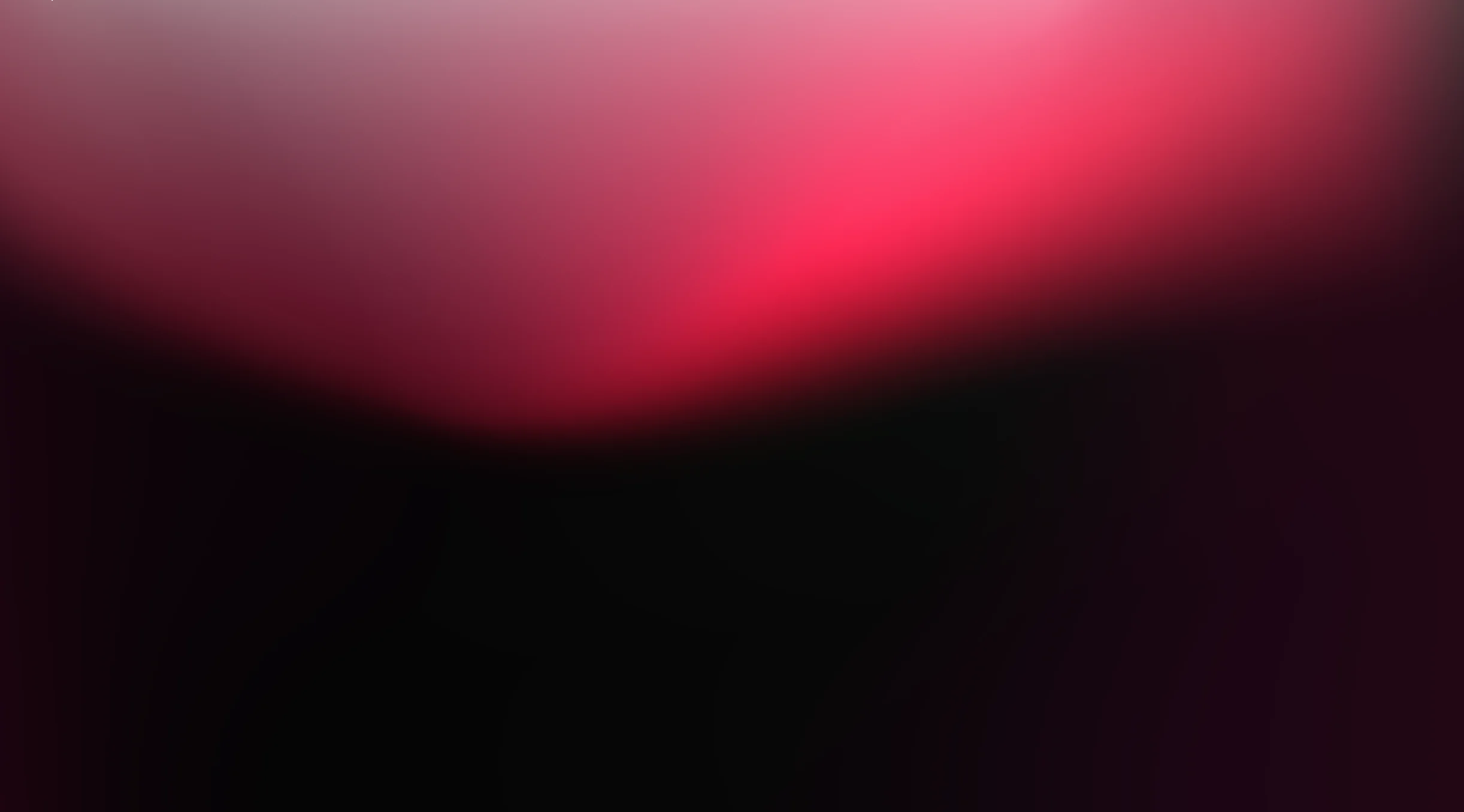
components
Input
v.1.0.0 | SaturnImport
1
import { Input } from '@kubit-ui-web/react-components';
Props
Let's delve into the versatility of the input component by examining all its avalaible props.
Uncontrolled
Property | Type | Default | Description |
---|---|---|---|
variant Required | String | - | Variant to add styles |
id | String | - | Id of the input |
overrideStyles | InputStylesProps | - | Styles that need to be overridden on the basic input |
error | Boolean | - | Specifies if the input element has error or not |
disabled | Boolean | - | Specifies if the input element is disabled or not |
value | String or Number | - | Value of the input |
errorExecution | ERROR_EXECUTION | - | When error handling occurs |
internalErrorExecution | INTERNAL_ERROR_EXECUTION | onChangeOnBlur | When internal error handling occurs |
keyValidation | String | - | Key validation to use in validationValue hook |
regex | String | - | Custom regex instead of maskType |
onInternalErrors | (errors: string) => void | - | Function that is called when an internal error occurs |
onError | (error: boolean) => void | - | Function that is called when an error occurs |
name | String | - | Specifies the name of the input element |
type | InputTypeType | TEXT | The type attribute specifies the type of input element to display. If the type attribute is not specified, the default type is text |
truncate | Boolean | false | Indicates if text value is truncated when is too big |
disableArrowUpDownInputNumber | Boolean | false | Disable arrows up and down when input type is number |
placeholder | String | - | Placeholder Input |
autocomplete | AUTOCOMPLETE_TYPE | undefined | Specifies whether an input field should have autocomplete on or off. Autocomplete allows the browser to predict the value. |
disableCopyAndPaste | Boolean | false | Disable copying and pasting |
maxLength | Number | - | Defines the maximum number of characters the user can enter into an input. This must be an integer value 0 or higher |
minLength | Number | - | Defines the minimum number of characters the user can enter into an input. This must be an integer value 0 or higher. If no minlength is specified, or an invalid value is specified, the input has no minimum length. |
min | Number | - | Specifies the minimum value for an input field. The min and max attributes work with the following input types: number, range, date, datetime-local, month, time and week. |
max | Number | - | Specifies the maximum value for an input field. The min and max attributes work with the following input types: number, range, date, datetime-local, month, time and week. |
ignoreKeys | String | - | Keys ignores in the onKeyDown function |
inputMode | InputModeType | text | InputMode for the input |
formatNumber | FormatNumber | - | Configuration for add thousand separators in input using Intl.NumberFormat (default locale `en-US`) (the HTML input element with type="number" does not support thousand separators). Internally we modify the type from input to text when the `formatNumber` prop exists. The value returned by the event is of type string. |
locale | String | - | Language of the specified date in the user agents timezone |
leftContent | String or JSX.Element | - | Prefix content |
leftExtraStyles | CSSProp | - | Styles needed for prefix content |
rightContent | String or JSX.Element | - | Suffix content |
rightExtraStyles | CSSProp | - | Styles needed for prefix content |
topExtraStyles | CSSProp | - | Styles needed for top content |
bottomExtraStyles | CSSProp | - | Styles needed for bottom content |
centerExtraStyles | CSSProp | - | Styles needed for center content |
extraAriaLabelledBy | String | '' | Allow to extend input "aria-labelledby" |
aria-label | String | - | Aria label of the input |
aria-labelledby | String | - | Aria labelledby of the input |
aria-describedby | String | - | Aria describedby of the input |
aria-invalid | Boolean | - | Aria invalid of the input |
aria-disabled | Boolean | - | Aria disabled of the input |
aria-expanded | Boolean | - | Indicates if a popover is expanded or collapsed. (Used for inputSearch and inputDropdown components) |
aria-haspopup | AriaHasPopupType | - | Indicates that the combobox opens a dialog. |
aria-controls | String | - | Identifies the element (or elements) whose contents or presence are controlled by the element on which this attribute is set. (Used for inputSearch component) |
role | ROLES | - | Prop used for accesibility to asign a rol |
mask | String | - | Mask input, is made with #, Example: ##-##-##. |
maskType | MASK_TYPE | - | Type of the mask |
onFocus | FocusEventHandler | - | Function that is called when focus on the component |
onBlur | FocusEventHandler | - | Function that is called when blur the component |
onChange | ChangeEventHandler | - | Function that is called when writting on the component |
onKeyDown | KeyboardEventHandler | - | Function that is triggered anytime the user presses a key on their keyboard |
onClick | MouseEventHandler | - | Function that is called when click on the component |
errorMessage | InputErrorMessageType | - | In the error state, the field is accompanied by a description of the problem to help resolve it. |
errorIcon | IElementOrIcon | - | In the error state, the field is accompanied by an error mbIcon and a description of the problem to help resolve it. |
errorAriaLiveType | AriaLiveOptionType | POLITE | Error message aria-live type |
helpMessage | InputHelpMessageType | - | Classic help text to give some more idea or condition about what is requested. |
informationAssociatedValue | InputInformationAssociatedValueType | - | In some cases, when the user enters data in the input, a (non-clickable) block with related information is displayed below, such as the name of the bank related to the account number. |
informationAssociatedButton | String | - | Add button for associated information |
textCounter | ReactNode | - | Element that allows to know the proximity to reach the limit of characters established for that input. The maximum number of characters will depend on the need of the flow, it should be customizable by property. |
required | Boolean | - | Required input option |
label | InputLabelType | - | Text of the input label. Indicates the information requested from the user. |
additionalInfo | ReactNode | - | This element is displayed to the right of the label secondary. |
secondaryLabel | ReactNode | - | This element is displayed to the right of the label when the input is not mandatory to filled. |
title | InputTitleType | - | Input title |
loading | Boolean | - | Show or not loader in input |
loader | ILoader | - | Loader component of the input |
icon Deprecated | IElementOrIcon | - | The input can contain an icon to help contextualize the user in relation to the information that is requested. |
iconPosition Deprecated | InputIconPosition | RIGHT | Set icon position |
rightIcon | IElemenOrIcon | - | Icon to show on the right side of the input. |
leftIcon | IElemenOrIcon | - | Icon to show on the left side of the input. |
dataTestId | String | - | Test id of the input. Internal components will concatenate from this test id |
ctv | InputStylesProps | - | Modify styles for the default version of the component |
Controlled
Property | Type | Default | Description |
---|---|---|---|
variant Required | String | - | Variant to add styles |
id | String | - | Id of the input |
overrideStyles | InputStylesProps | - | Styles that need to be overridden on the basic input |
name | String | - | Specifies the name of the input element |
type | InputTypeType | TEXT | The type attribute specifies the type of input element to display. If the type attribute is not specified, the default type is text |
truncate | Boolean | false | Indicates if text value is truncated when is too big |
disableArrowUpDownInputNumber | Boolean | false | Disable arrows up and down when input type is number |
placeholder | String | - | Placeholder Input |
autocomplete | AUTOCOMPLETE_TYPE | undefined | Specifies whether an input field should have autocomplete on or off. Autocomplete allows the browser to predict the value. |
disableCopyAndPaste | Boolean | false | Disable copying and pasting |
maxLength | Number | - | Defines the maximum number of characters the user can enter into an input. This must be an integer value 0 or higher |
minLength | Number | - | Defines the minimum number of characters the user can enter into an input. This must be an integer value 0 or higher. If no minlength is specified, or an invalid value is specified, the input has no minimum length. |
min | Number | - | Specifies the minimum value for an input field. The min and max attributes work with the following input types: number, range, date, datetime-local, month, time and week. |
max | Number | - | Specifies the maximum value for an input field. The min and max attributes work with the following input types: number, range, date, datetime-local, month, time and week. |
ignoreKeys | String | - | Keys ignores in the onKeyDown function |
inputMode | InputModeType | text | InputMode for the input |
formatNumber | FormatNumber | - | Configuration for add thousand separators in input using Intl.NumberFormat (default locale `en-US`) (the HTML input element with type="number" does not support thousand separators). Internally we modify the type from input to text when the `formatNumber` prop exists. The value returned by the event is of type string. |
locale | String | - | Language of the specified date in the user agents timezone |
leftContent | String or JSX.Element | - | Prefix content |
leftExtraStyles | CSSProp | - | Styles needed for prefix content |
rightContent | String or JSX.Element | - | Suffix content |
rightExtraStyles | CSSProp | - | Styles needed for prefix content |
topExtraStyles | CSSProp | - | Styles needed for top content |
bottomExtraStyles | CSSProp | - | Styles needed for bottom content |
centerExtraStyles | CSSProp | - | Styles needed for center content |
extraAriaLabelledBy | String | '' | Allow to extend input "aria-labelledby" |
aria-label | String | - | Aria label of the input |
aria-labelledby | String | - | Aria labelledby of the input |
aria-describedby | String | - | Aria describedby of the input |
aria-invalid | Boolean | - | Aria invalid of the input |
aria-disabled | Boolean | - | Aria disabled of the input |
aria-expanded | Boolean | - | Indicates if a popover is expanded or collapsed. (Used for inputSearch and inputDropdown components) |
aria-haspopup | AriaHasPopupType | - | Indicates that the combobox opens a dialog. |
aria-controls | String | - | Identifies the element (or elements) whose contents or presence are controlled by the element on which this attribute is set. (Used for inputSearch component) |
role | ROLES | - | Prop used for accesibility to asign a rol |
mask | String | - | Mask input, is made with #, Example: ##-##-##. |
maskType | MASK_TYPE | - | Type of the mask |
onFocus | FocusEventHandler | - | Function that is called when focus on the component |
onBlur | FocusEventHandler | - | Function that is called when blur the component |
onChange | ChangeEventHandler | - | Function that is called when writting on the component |
onKeyDown | KeyboardEventHandler | - | Function that is triggered anytime the user presses a key on their keyboard |
onClick | MouseEventHandler | - | Function that is called when click on the component |
errorMessage | InputErrorMessageType | - | In the error state, the field is accompanied by a description of the problem to help resolve it. |
errorIcon | IElementOrIcon | - | In the error state, the field is accompanied by an error mbIcon and a description of the problem to help resolve it. |
errorAriaLiveType | AriaLiveOptionType | POLITE | Error message aria-live type |
helpMessage | InputHelpMessageType | - | Classic help text to give some more idea or condition about what is requested. |
informationAssociatedValue | InputInformationAssociatedValueType | - | In some cases, when the user enters data in the input, a (non-clickable) block with related information is displayed below, such as the name of the bank related to the account number. |
informationAssociatedButton | String | - | Add button for associated information |
textCounter | ReactNode | - | Element that allows to know the proximity to reach the limit of characters established for that input. The maximum number of characters will depend on the need of the flow, it should be customizable by property. |
required | Boolean | - | Required input option |
label | InputLabelType | - | Text of the input label. Indicates the information requested from the user. |
additionalInfo | ReactNode | - | This element is displayed to the right of the label secondary. |
secondaryLabel | ReactNode | - | This element is displayed to the right of the label when the input is not mandatory to filled. |
title | InputTitleType | - | Input title |
loading | Boolean | - | Show or not loader in input |
loader | ILoader | - | Loader component of the input |
icon Deprecated | IElementOrIcon | - | The input can contain an icon to help contextualize the user in relation to the information that is requested. |
iconPosition Deprecated | InputIconPosition | RIGHT | Set icon position |
rightIcon | IElemenOrIcon | - | Icon to show on the right side of the input. |
leftIcon | IElemenOrIcon | - | Icon to show on the left side of the input. |
dataTestId | String | - | Test id of the input. Internal components will concatenate from this test id |
ctv | InputStylesProps | - | Modify styles for the default version of the component |