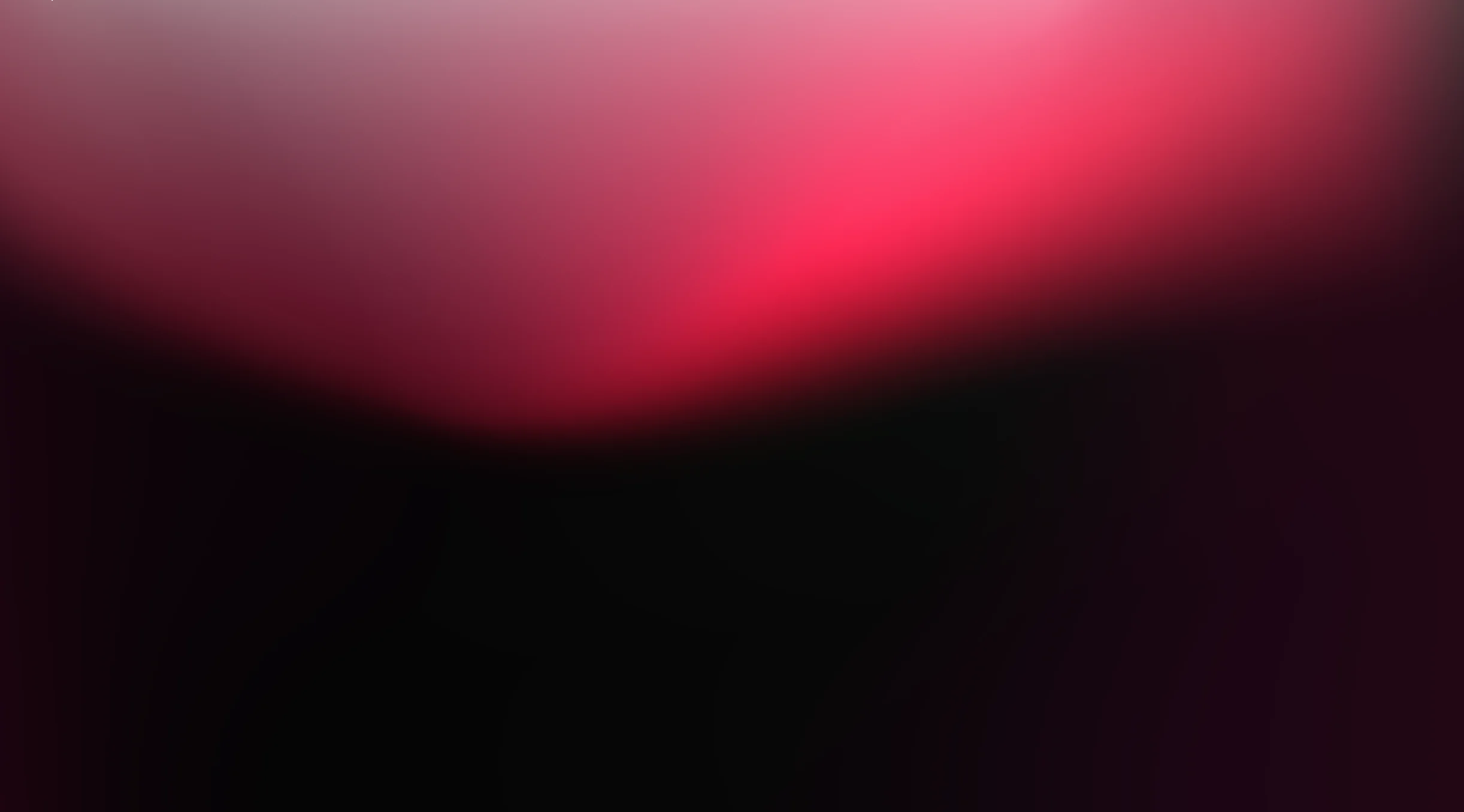
components
Table
v.1.0.0 | SaturnIt displays data collections grouped into a table structure.
<Table
variant="DEFAULT"
captionDescription="Table caption"
aria-label="ariaLabel table"
headerVariant="PRIMARY"
formatListInMobile={true}
headers={[
{ id: 'date', label: 'Date', config: {} },
{ id: 'accountNumber', label: 'Account number', config: {} },
]}
Variant
In Kubit Design System, users have the freedom to generate variants for each component according to their needs. While predefined variants are provided as examples, they can be modified or new ones can be added to align with the specific requirements of each project.
To manage the visual appearance of the table, use the props variant, headerVariant and rowVariant (inside each values prop).
- variant: Customize the visual appearance of the table component.
- headerVariant: Customize the visual appearance of the table header.
- rowVariant: Customize the visual appearance of the table row (default variant is DEFAULT).
<Table
variant="DEFAULT"
captionDescription="Table caption"
aria-label="ariaLabel table"
headerVariant="PRIMARY"
formatListInMobile={true}
headers={[
{ id: 'date', label: 'Date', config: {} },
{ id: 'accountNumber', label: 'Account number', config: {} },
]}
Custom values
In order to customize the values of the table you can use the value header prop.
<Table variant='DEFAULT' captionDescription='Table caption' aria-label='ariaLabel table' headerVariant='PRIMARY' formatListInMobile={true} headers={ [ {id: 'date', label: 'Date', config: {}}, {id: 'accountNumber', label: 'Account number', config: {}},
List format when mobile
In order to display the table as a list in mobile, use the formatListInMobile prop (default false).
<Table
variant="DEFAULT"
captionDescription="Table caption"
aria-label="ariaLabel table"
headerVariant="PRIMARY"
formatListInMobile={true}
headers={[
{ id: 'date', label: 'Date', config: {} },
{ id: 'accountNumber', label: 'Account number', config: {} },
]}
Custom alignment
Configure alignment through the alignHeader and alignValue header config props
<Table
variant='DEFAULT'
captionDescription='Table caption'
aria-label='ariaLabel table'
headerVariant='PRIMARY'
headers={
[
{id: 'date', label: 'Date', config: {alignHeader: 'left', alignValue: 'left'}},
{id: 'accountNumber', label: 'Account number', config: {alignHeader: 'right', alignValue: 'right'}}
]
Custom width
Configure width through the width and flexWidth header config prop
<Table
variant='DEFAULT'
captionDescription='Table caption'
aria-label='ariaLabel table'
headerVariant='PRIMARY'
headers={
[
{id: 'date', label: 'Date', config: {width: '25%', alignHeader: 'left', alignValue: 'left'}},
{id: 'description', label: 'Description', config: {width: '75%', alignHeader: 'left', alignValue: 'left'}}
]
Hide column on breakpoint
Hide a column on a specific breakpoint using the hidden header config prop.
<Table variant='DEFAULT' captionDescription='Table caption' aria-label='ariaLabel table' headerVariant='PRIMARY' headers={ [ {id: 'date', label: 'Date', config: {alignHeader: 'left', alignValue: 'left'}}, {id: 'hidden', label: 'Hidden', config: {hidden: {["TABLET"]: true, ["MOBILE"]: true}}}, {id: 'accountNumber', label: 'Account number', config: {alignHeader: 'right', alignValue: 'right'}}
Hide header on breakpoint
Hide table header on a specific breakpoint using the hiddenHeaderOn prop.
<Table
variant='DEFAULT'
captionDescription='Table caption'
aria-label='ariaLabel table'
hiddenHeaderOn={{'TABLET': true, 'MOBILE': true}}
headerVariant='PRIMARY'
headers={
[
{id: 'description', label: 'Hiden on mobile and tablet', config: {}},
]
Divider
In order to add a divider between the values of the table, you can configure divider as lineSeparator or as divider component.
Divider as Line Separator component
In order to configure a lineSeparator divider, you can set the hasDivider prop in the associated header config prop.
<Table
variant="DEFAULT"
captionDescription="Table caption"
aria-label="ariaLabel table"
headerVariant="PRIMARY"
formatListInMobile={true}
headers={[
{ id: 'date', label: 'Date', config: { hasDivider: true } },
{ id: 'name', label: 'Name', config: { alignHeader: 'left', alignValue: 'left' } },
{ id: 'accountNumber', label: 'Account number', config: { alignHeader: 'right', alignValue: 'right' } },
Divider as Divider component
In order to configure a divider separator, you can set the dividerContent prop in the associated value config prop. This prop receives:
- leftLabel: The left label of the divider.
- rightLabel: The right label of the divider.
- leftSublabel: The left sublabel of the divider.
- rightSublabel: The right sublabel of the divider.
- icon: The icon of the divider.
- iconTooltip: Tooltip data that will be displayed with the icon of the divider.
<Table variant="DEFAULT" captionDescription="Table caption" ariaLabelTable="ariaLabel table" headerVariant="PRIMARY" formatListInMobile={true} headers={[ { id: 'date', label: 'Date', config: { hasDivider: true } }, { id: 'name', label: 'Name', config: { alignHeader: 'left', alignValue: 'left' } }, { id: 'accountNumber', label: 'Account number', config: { alignHeader: 'right', alignValue: 'right' } },
Expanded content
The table can have an accordion behaviour if accordionIcon is added. Also, the initialExpanded prop can be set to indicate if the component is initially expanded. Into values prop, the expandedContent prop can be added to display the content when the accordion is expanded.
<Table variant='DEFAULT' headerVariant='PRIMARY' expandedContentHelpMessage='Can expant the row to see more information' accordionIcon={{icon: "ICON_PLACEHOLDER"}} formatListInMobile={true} headers={ [ {id: 'date', label: 'Date', config: { alignHeader: 'left', alignValue: 'left' }}, {id: 'accountNumber', label: 'Account number', config: { alignHeader: 'right', alignValue: 'right' }}
Footer
A footer can be added to the end of the table using the footer prop. Check the specific component documentation for more information.
Use simpleContainer prop from footer to set the footer as a div instead of a footer HTML element.
<div style={{ width: '100%' }}> <Table variant="DEFAULT" captionDescription="Table caption" aria-label="ariaLabel table" headerVariant="PRIMARY" headers={[ { id: 'date', label: 'Date', config: {} }, { id: 'accountNumber', label: 'Account number', config: {} }, ]}